Session Management - Backend Server
Learn how to manage sessions with a backend server.
Managing Sessions With a Backend Server
After a user authenticates, you'll typically want to create a session to persist the user's authenticated state across requests between the browser and your web application. For web applications using a backend server, there are generally two approaches for managing sessions: client-side sessions and server-side sessions.
Client-Side Sessions
Client-side sessions are where all of the session data is stored inside a session cookie. This approach is useful if you don't want to worry about storing session data in an external datastore. Before the session data is stored in the cookie, it must be encrypted. Encrypting the cookie contents prevents sensitive data from being exposed through the cookie and also stops unwanted tampering with the cookie's value.
One limitation of this approach is that most browsers enforce a max cookie size of 4 KBs, so if your session data exceeds this size, you'll need to split the data across multiple cookies or use server-side sessions. Also, with client-side sessions, it's not possible for an external party to immediately revoke a user's session since they don't have access to the session cookie. To work around this limitation, it's advisable to include the access and refresh tokens in the session cookie. When validating the session cookie on the server, the access token expiration time should be verified to ensure that it hasn't expired. If the access token has expired and can't be refreshed using the refresh token, then the session should be considered invalid. By utilizing this approach, the user's session can be indirectly invalidated by revoking the refresh token; however, it's important to note that there could be a delay between when the refresh token is revoked and when the session is invalidated, based on how long it takes for the access token to expire. Therefore, it's best to use short-lived access tokens to minimize this delay.
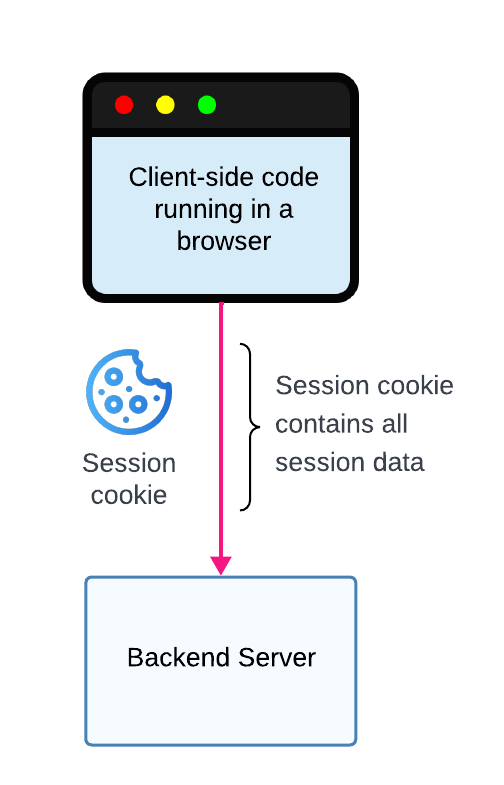
Client-side sessions
Server-Side Sessions
Server-side sessions are where all the session data is stored in an external datastore (such as Redis), and only the session ID is stored within the session cookie. When the server receives the session cookie, it uses the session identifier contained within the cookie to look up the complete session information from the external datastore. Server-side sessions are useful for applications with large sessions that can't fit within the 4KB size limit of cookies. Also, server-side sessions provide more control over session revocation since the sessions can easily be removed from the external datastore to invalidate the session. The main downside to using server-side sessions is that they add extra latency to each request to look up the session information from the external datastore. Furthermore, server-side sessions are also more complicated to implement since they require setting up an external datastore to persist the sessions.
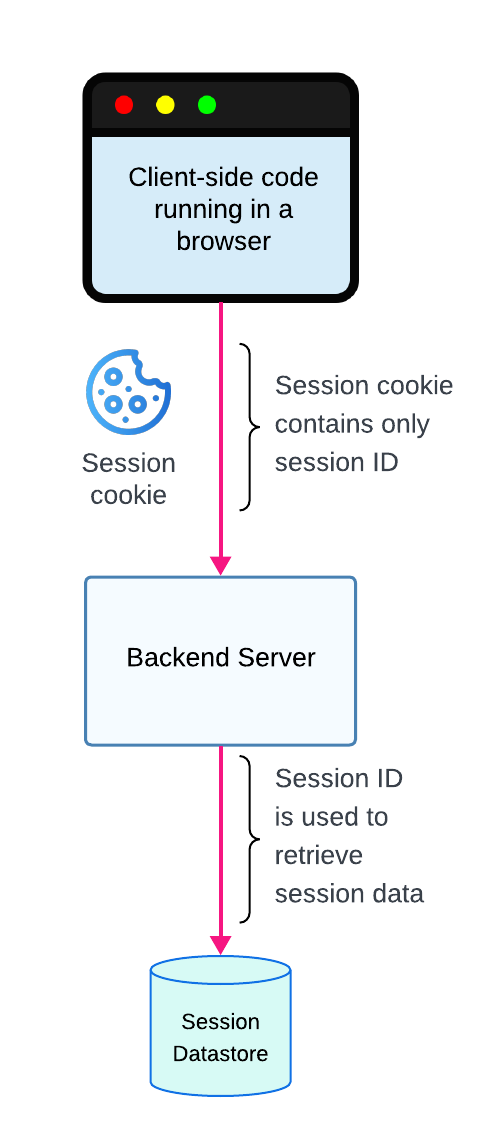
Server-side sessions
Securing the Session Cookie
Whether you're using client-side or server-side sessions, you should always set the following cookie attributes in order to secure the session cookie:
- HttpOnly - Ensures that the cookie can't be accessed by JavaScript running in the browser. This helps protect against cross-site scripting (XSS) attacks.
- SameSite - The SameSite attribute should be set to a value of Strict or Lax. This will stop the cookie from being sent during cross-site requests, helping to prevent cross-site request forgery (CSRF) attacks.
- Secure - Ensures that cookies are only sent to the server over HTTPS.
Terminating Sessions on Logout
When an authenticated user logs out of your application, the application must ensure that all authenticated state associated with the user is cleaned up. Typically, three items need to be deleted in order to log the user out properly: The user's Application Session, refresh token, and Wristband Auth Session.
Deleting the Application Session
Regardless of whether the session is managed client-side or server-side, the backend server must delete the application's session cookie. Deletion of the session cookie can be done by setting the max-age
attribute to 0 and setting the cookie's value to an empty string. Additionally, the corresponding session data should be removed from the external datastore if server-side sessions are used.
Revoking the Refresh Token
If the session has a refresh token associated with it, then the application should call the Wristband Revoke Token API to revoke the refresh token.
Deleting the Wristband Auth Session
Lastly, the application should redirect to the Wristband Logout API, which will cause the Wristband Auth Session to be deleted.
Accessing Session Data From Client-Side JavaScript
Typically, you'll need to expose the user's session data to your JavaScript code running in the browser; for example, to populate the authentication context in your React application. To do this, you'll need to create a Session Endpoint on your backend server that will use the contents of the session cookie to derive the user's session data and then return it in the response. Your client-side JavaScript code can then call the Session Endpoint to get access to the user's session data.
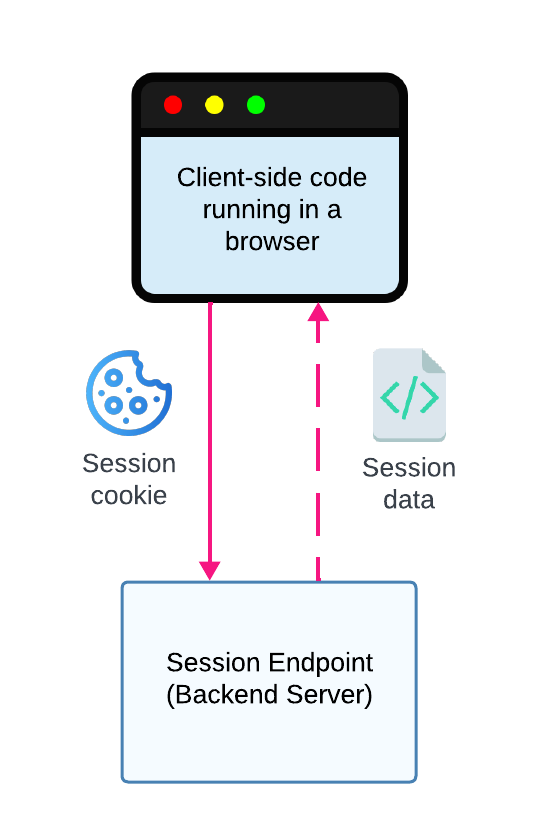
Client-side JavaScript calls the Session Endpoint to retrieve the user's session data.
Updated 15 days ago