CSRF Protection - Backend Server
Learn how to secure backend servers with CSRF tokens to protect against cross-site request forgery attacks.
What is Cross-Site Request Forgery (CSRF)?
Cross Site Request Forgery (CSRF) is a security vulnerability where attackers trick authenticated users into unknowingly submitting malicious requests to your application. Since these actions are performed within the context of a user’s session, they may lead to unintended changes or data exposure without the user’s consent.
CSRF Prevention Methods
For detailed guidance on CSRF protection techniques and best practices, always refer to the OWASP CSRF Prevention Cheat Sheet. OWASP outlines several tactics for defending against CSRF attacks. Among these, the signed double-submit cookie pattern is the recommended choice for minimizing the risk of interception or forgery.
Implementing the Signed Double-Submit Cookie Pattern
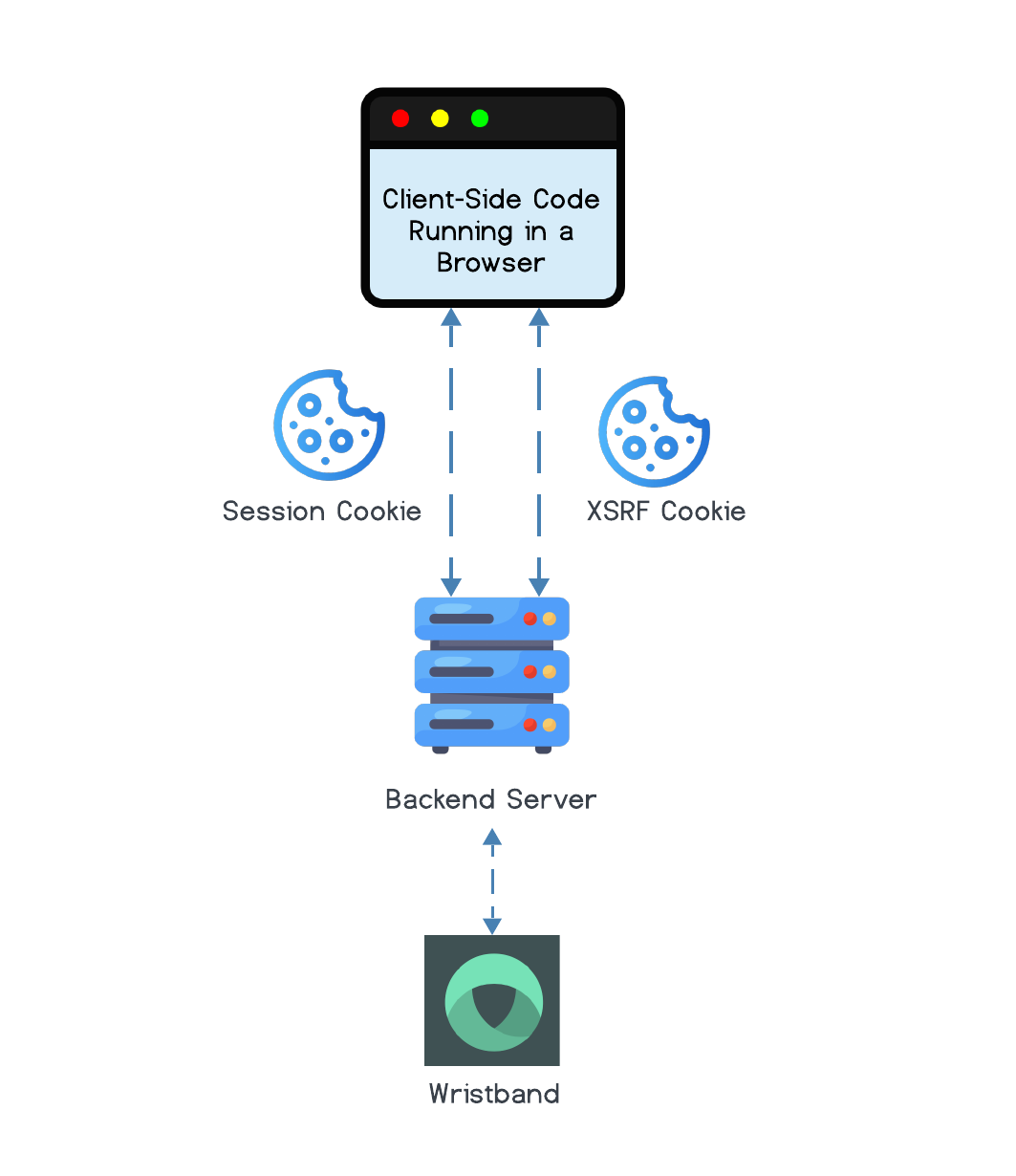
Backend Server Integration with CSRF Cookie
For implementing CSRF protection in a backend server integration, we recommend a structured approach to implementing the signed double-submit cookie pattern. Here’s an overview of how it looks in the context of a Wristband integration:
- Authorization Callback & Token Acquisition: After the user successfully authenticates through the Wristband login flow, they are redirected to your backend server’s callback endpoint, where the authorization code is exchanged for access and refresh tokens.
- CSRF Secret Creation: While still in the Callback Endpoint, the server generates a CSRF secret and securely stores it in the user’s application session.
- Signed CSRF Token Generation: Using the CSRF secret, the server generates a signed CSRF token, which is sent to the client as a non-HttpOnly cookie alongside the session cookie. This allows client-side JavaScript to access the CSRF token and include it in subsequent requests for validation.
- Client Requests with CSRF Validation: For subsequent requests, the client-side JavaScript sends a custom request header containing the CSRF token. This ensures each request is validated with both the session and CSRF tokens, protecting against unauthorized actions.
- Server-Side CSRF Middleware Validation: The server's middleware verifies that the CSRF token in the custom request header was cryptographically generated from the CSRF secret in your application session. If that is not case, then the server returns an unauthorized error.
- Token Regeneration on Each Request: Upon successful validation, the middleware regenerates a new CSRF token using the CSRF secret in the session and sends it as a refreshed CSRF cookie with each response.
- CSRF Token Cleanup on Logout: When the user logs out, the logout endpoint on the server clears both the session and the CSRF cookies to ensure that no stale tokens remain.
Updated 28 days ago