🧪 Test CSRF Middleware
Test that your CSRF middleware is protecting your application.
Tests not working?
If you get stuck, contact us and our development team will work directly with you to get unblocked.
After implementing the Synchronizer Token pattern, let's verify that it's working correctly. The following tests will ensure that the CSRF token is created during login, that your CSRF middleware verifies the CSRF token for authenticated endpoints, and that the CSRF cookie is cleaned up after logout.
1. Verify CSRF Token is Created During Login
After a user successfully authenticates, Wristband will redirect to your application's Callback Endpoint. The Callback Endpoint should then create a CSRF-TOKEN
cookie containing the CSRF token. Also, when your application loads and calls the Session Endpoint, you should see the CSRF token getting passed in the X-Csrf-Token
request header.
Test Steps
- Open a browser and set up the browser's dev tools to actively monitor network requests. Also, ensure that network requests are configured to be preserved so you can examine them afterward.
- Navigate to your application's Tenant Login Page.
- Complete the authentication flow.
Verification Checks
-
In your browser's developer tools, find the network request to your application's Callback Endpoint. Verify in the network request that you see the
Set-Cookie
response header containing theCSRF-TOKEN
.
-
Verify the
CSRF-TOKEN
Cookie appears in your browser's storage.
-
In your browser's developer tools, find the network request to your application's Session Endpoint. Verify in the network request that you see the
X-Csrf-Token
request header being set.
-
Verify that the Session Endpoint returns a 200 response.
2. Verify Requests With Invalid CSRF Tokens Fail
Now that we've tested that the happy path works, let's try deleting the CSRF-TOKEN
cookie so that the X-Csrf-Token
request headers will no longer contain a valid CSRF token. Requests to the Session Endpoint should now fail with a 403 Forbidden
response.
Test Steps
-
While still logged in to the application, manually delete the CSRF cookie from the browser.
-
Refresh your application to trigger a call to the Session Endpoint.
Verification Checks
-
In your browser's developer tools, find the network request to your application's Session Endpoint. Verify that the response is now a 403.
-
Verify that after receiving the 403 response, you are redirected to your application's Login Endpoint.
3. Verify CSRF Cookie Removal on Logout
Finally, let's make sure the CSRF cookie gets removed from the browser when a user logs out.
Test Steps
- Ensure that you are logged into your application and can see that the
CSRF-TOKEN
cookie is stored in your browser. In the previous test, we deleted this cookie, so you'll probably need to log in again to recreate it. - Update the browser's address bar to navigate to your application's Logout Endpoint (e.g. http://localhost:3000/auth/logout). This will cause the logout flow to execute.
Verification Checks
-
In your browser's developer tools, find the network request to your application's Logout Endpoint. Verify in the network request that you can see the Set-Cookie response header containing a cookie named
CSRF-TOKEN
. The cookie's value should be blank, and itsMax-Age
set to 0 in order to delete the cookie from the browser.
- Check your browser's cookie storage to ensure that there is no longer a
CSRF-TOKEN
cookie associated with your application's domain.
If all tests succeed, then CSRF protection has been successfully implemented. 🎉
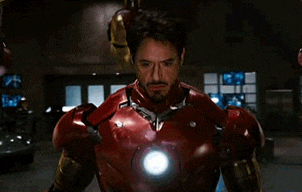
Well done. You've got the ultimate defense now.
Updated 13 days ago