Authentication - Backend Server
A high-level overview of how authentication works with a backend server.
Login
Steps:
- The user clicks the Login button on your website or application.
- The user is sent to the Login Endpoint implemented by your backend server.
- The backend server creates an Authorization Request and redirects the user to the Wristband Authorize Endpoint.
- Wristband validates and records the Authorization Request and redirects the user to the Wristband-hosted Login page.
- The user provides their credentials to authenticate.
- The Login Page redirects to your application's Callback Endpoint with an authorization code.
- The Callback Endpoint calls Wristband's Token Endpoint to exchange the authorization code for an access token.
- The Callback Endpoint establishes an application session and sets a session cookie.
- The user is redirected to your application's entry point.
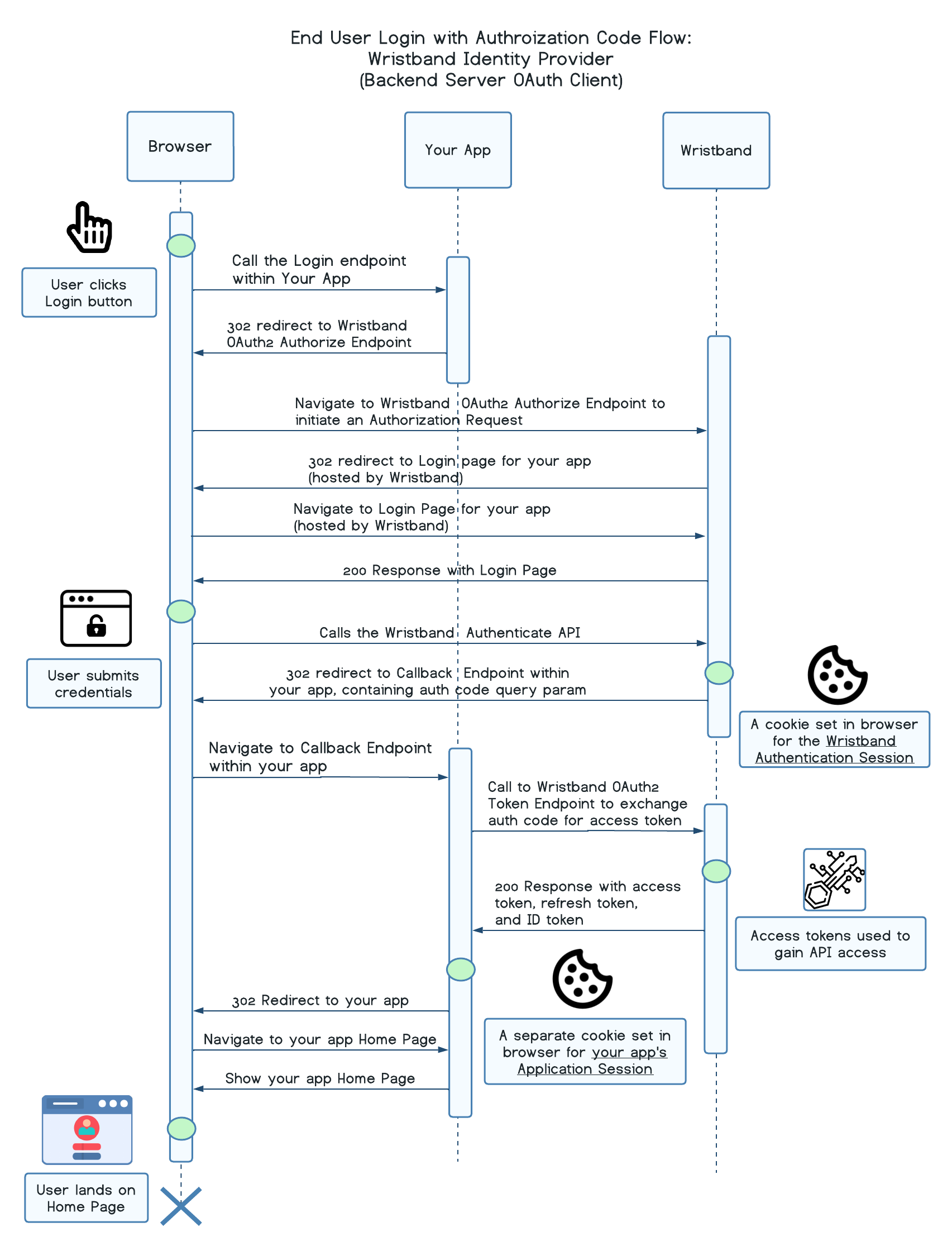
Login Flow for Backend Server Integration
Logout
Steps:
- The user clicks the Logout button within your application.
- The user is sent to the Logout endpoint of your backend server.
- The backend server destroys the session cookie.
- The backend server calls the Revoke Token Endpoint to revoke the refresh token.
- The user is redirected to the Wristband Logout Endpoint.
- Wristband destroys the authentication session and redirects the user to your application's Login endpoint.
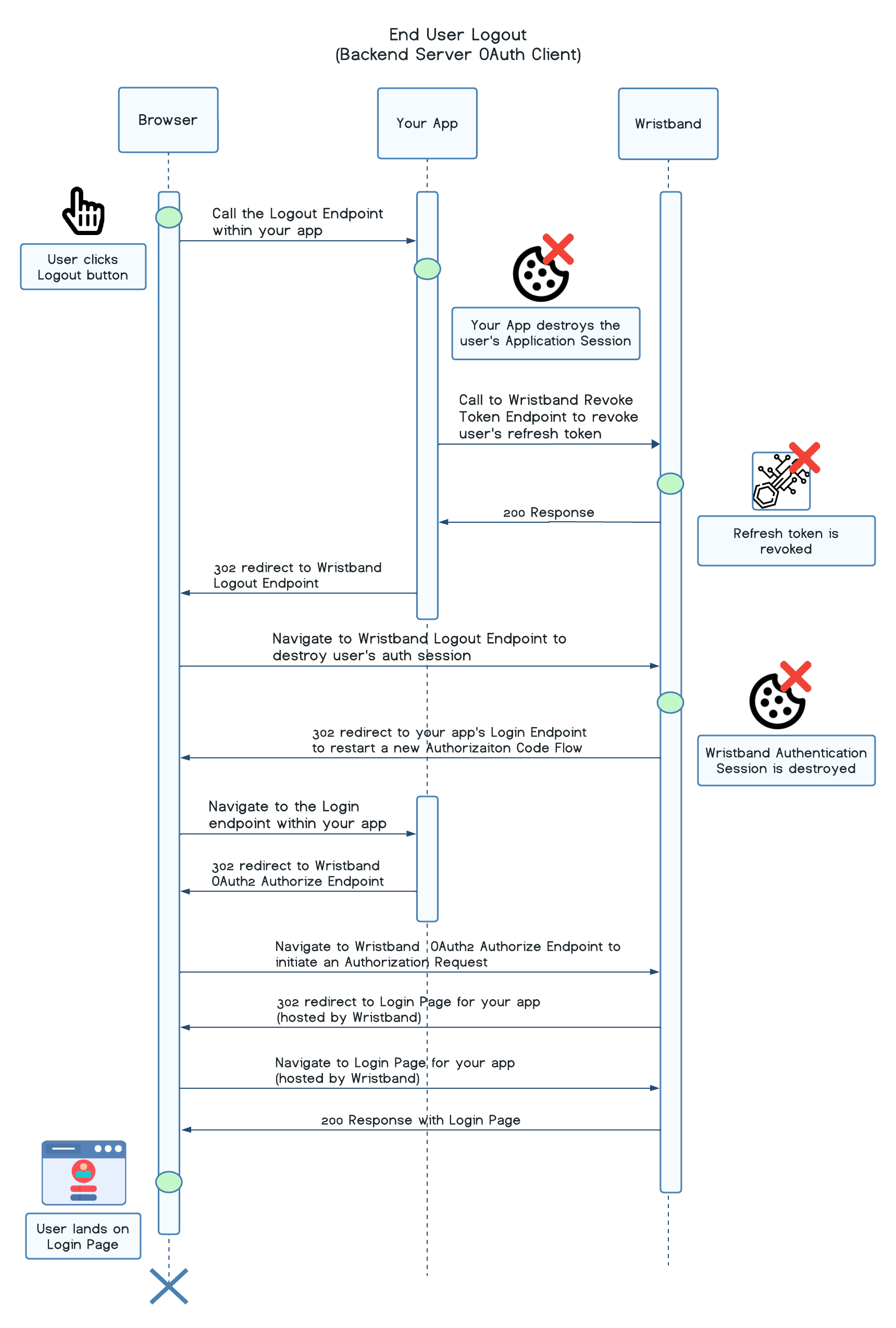
Logout Flow for Backend Server Integration
Refreshing Access Tokens
Steps:
- The client-side application sends a request to any protected API on the backend server. The session cookie is automatically included in the request headers.
- Before reaching the business logic of the requested route, an authentication middleware processes the request. The middleware accesses the user's session data, which includes the access token, refresh token, and token expiration time. The session data is accessed as follows:
- For Cookie Sessions: The middleware decrypts the encrypted cookie value to retrieve the session data.
- For Server Sessions with a Session Store: The middleware looks up the session data in the backend session store using the session ID provided in the cookie.
- The middleware checks if the access token is expired. If the access token is expired, the middleware makes a request to Wristband's Token Endpoint. The request includes the refresh token from the user's session in the request body.
- Wristband verifies the refresh token's validity. If valid, it generates a new access token and includes it in the response to the backend server.
- After obtaining a valid access token, the middleware allows the protected API to proceed with its business logic. Once the API finishes processing, the session is updated:
- For Cookie Sessions: The new access token and refresh token are embedded in the session data. The session data is encrypted and sent back as the new cookie value.
- For Server Sessions with a Session Store: The new tokens are persisted in the session store, and if applicable, the TTL of the session store record is updated to reflect the new expiration time.
- The session cookie’s max age and/or expiration time are updated and sent back to the client as part of the API response headers.
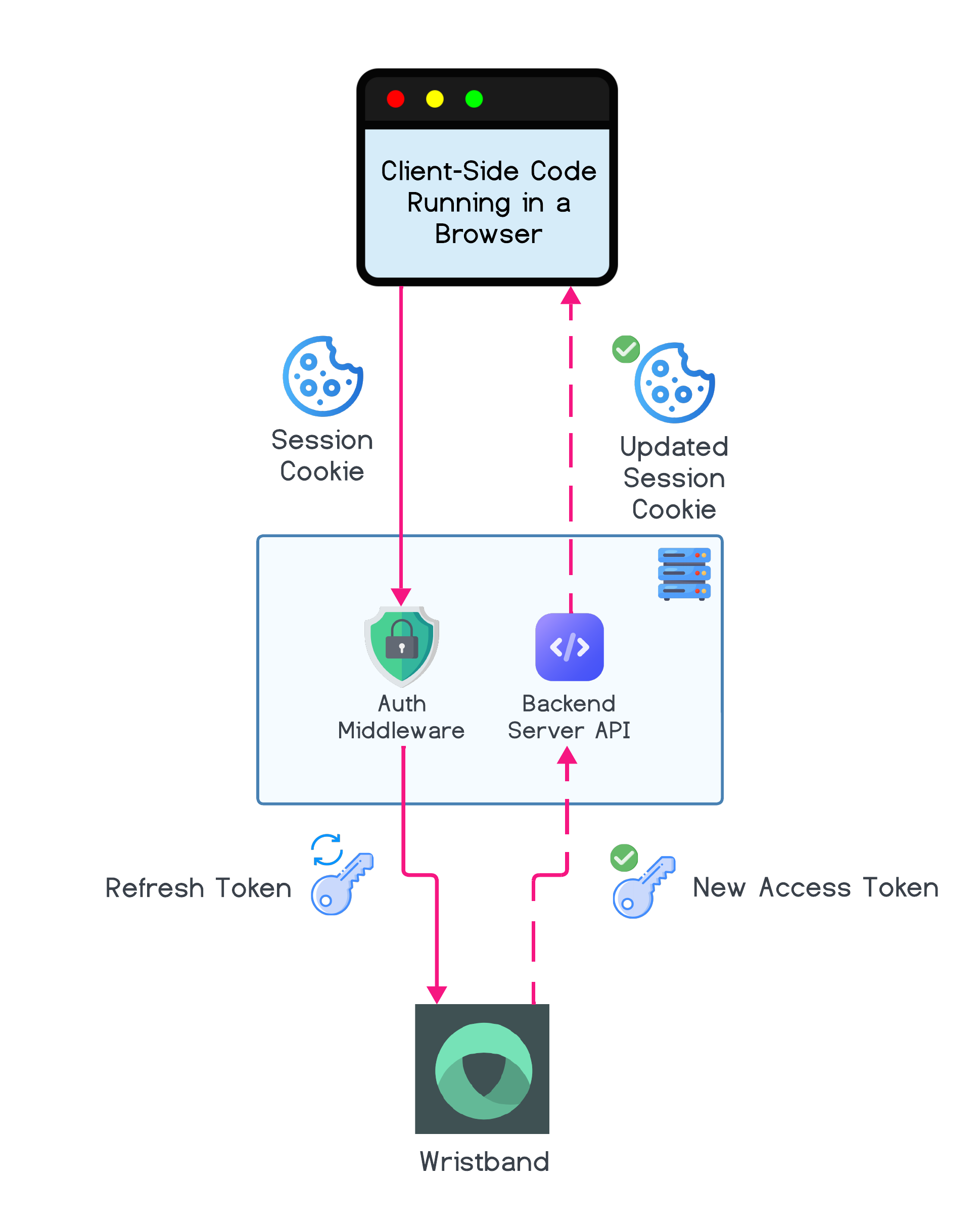
Refresh Token Flow for Backend Server Integration
Additional Considerations
Access tokens should be refreshed slightly before their expiration time is reached in order to prevent situations where the access token expires while being processed by downstream API servers, resulting in a 401 being returned to the app. While the app should be able to recover from the 401 by refreshing the access token and retrying the request, it results in extra latency due to having to repeat the same request.
Refresh Token Rotation
Wristband supports refresh token rotation, a feature that enhances security by issuing a new refresh token with every use, reducing the risk of token replay attacks. However, backend servers inherently provide a secure location for storing tokens, as they do not expose tokens to malicious javascript running in the browser. This minimizes the risk of token theft or misuse.
For this reason, refresh token rotation is not required for backend server integrations. Tokens remain secure as long as proper storage and access controls are in place on the backend. To simplify implementation and avoid unnecessary complexity, Wristband disables refresh token rotation by default for backend server configurations.
Handling Refresh Failures
The token refresh operation can fail for the following reasons:
- The refresh token’s expiration time has been exceeded.
- The refresh token has been revoked.
- The refresh token has been tampered with and is no longer valid.
- An invalid request, such as incorrect client credentials.
- Network issues or server errors (e.g.:
500 Internal Server Error
).
Retrying Refresh Requests
For transient issues like network or server errors, it is advised to retry the refresh request to minimize disruption for the end user. We recommend implementing retry logic with a fixed or exponential backoff.
Server-side Error Handling
When Wristband’s Token Endpoint returns an error and all retries fail, the backend server should perform the following actions.
- Invalidate the user's session by doing the following:
- Cookie-based sessions: Clear the session cookie and set it to expire immediately.
- Server-side sessions: Clear the session cookie and set it to expire immediately. In addition, remove the session data from the backend session store.
- Return an appropriate error response to the client. The error response should use a
401 Unauthorized
status code to indicate that the user is no longer authenticated.
Client-side Error Handling
When the client-side application receives a 401 response from the server, you can handle it in various ways based on your UX and security requirements:
- Redirect to Login Without Destroying the Wristband Auth Session: Send the user to the Wristband login page without calling the Wristband logout endpoint. If the user still has a valid Wristband auth session, they will automatically be re-authenticated without having to enter their credentials.
- Full Logout and Re-authentication: Clean up any local session data from the client-side application, then redirect the user to Wristband’s Logout Endpoint to terminate the Wristband auth session. This will force the user to re-authenticate the next time they try to access your application.
Updated 3 months ago