Authentication - Native
A high-level overview of how authentication works with a native mobile or desktop app.
Login
Steps:
- The user clicks the Login button within your native application.
- The native app creates an Authorization Request and sends the user to a browser for redirection to the Wristband Authorize Endpoint.
- Wristband validates and records the Authorization Request and redirects the user to the Wristband-hosted Login page.
- The user provides their credentials to authenticate.
- The Login Page redirects back to the native app's Callback Endpoint with an authorization code via deep linking.
- The Callback Endpoint calls Wristband's Token Endpoint to exchange the authorization code for an access token.
- The Callback Endpoint stores the access token and refresh token in secure storage.
- The user is redirected to your native app's entry point.
Logout
Steps:
- The user clicks the Logout button within your native app.
- The app invokes a
logout()
function, destroying tokens in secure storage. - The app calls the Revoke Token Endpoint to revoke the refresh token.
- The user is redirected to the Wristband Logout Endpoint.
- Wristband destroys the authentication session and redirects the user to your app's Login screen via deep linking.
Refreshing Access Tokens
There are a couple of approaches your native application can take to handle refreshing tokens:
Approach | How it works | Advantages | Limitations |
---|---|---|---|
Refreshing on API Requests | The native app checks if the access token is near expiration before API calls, refreshing it if needed before proceeding with the request. | Minimizes unnecessary refreshes and is simple to implement. | The first API request after a period of inactivity may lag due to the token being refreshed. Need to implement locking to ensure concurrent API requests don't trigger token refreshes simultaneously. |
Using a background timer to trigger token refreshes | The native app uses a timer to schedule a token refresh before the access token expires. | Mitigates the extra latency incurred by performing a token refresh on API calls. | Adds unnecessary refreshes during inactivity. Timers add complexity to track token expiry. |
Best Practice: Combine Both Strategies
You can also combine both strategies to get the best of both worlds. Combining both strategies ensures tokens are refreshed during idle periods while outgoing checks handle edge cases like missed timers or unexpected expiration.
For many native apps, only refreshing during outgoing requests is sufficient and simplifies the implementation. However, using a timer to refresh tokens in the background becomes valuable for applications that want to ensure latency isn't affected by token refreshes during outbound API calls. The choice ultimately depends on your application’s needs.
Below are the steps that an application should take to handle refreshing tokens before an API request:
- Before the native app calls a protected API, it first checks whether the access token has expired using the
expiresIn
value received from Wristband's Token Endpoint. - If the access token has expired or is near expiration, the native app makes a request to Wristband's Token Endpoint to refresh the access token. The request includes the refresh token in the request body.
- Wristband verifies the refresh token's validity. If valid, it generates a new access token and includes it in the response to the app.
- The app securely stores the updated tokens in the platform's secure storage mechanism (e.g.: Keychain for iOS).
- The native app retries the original API request using the refreshed access token in the Authorization header (e.g.:
Authorization: Bearer <access_token>
).
Access Token Expiry Monitoring
The native app can optionally track token validity using the newest expiresIn value and refresh proactively by setting a timer to refresh the token independently of other API requests just before expiration.
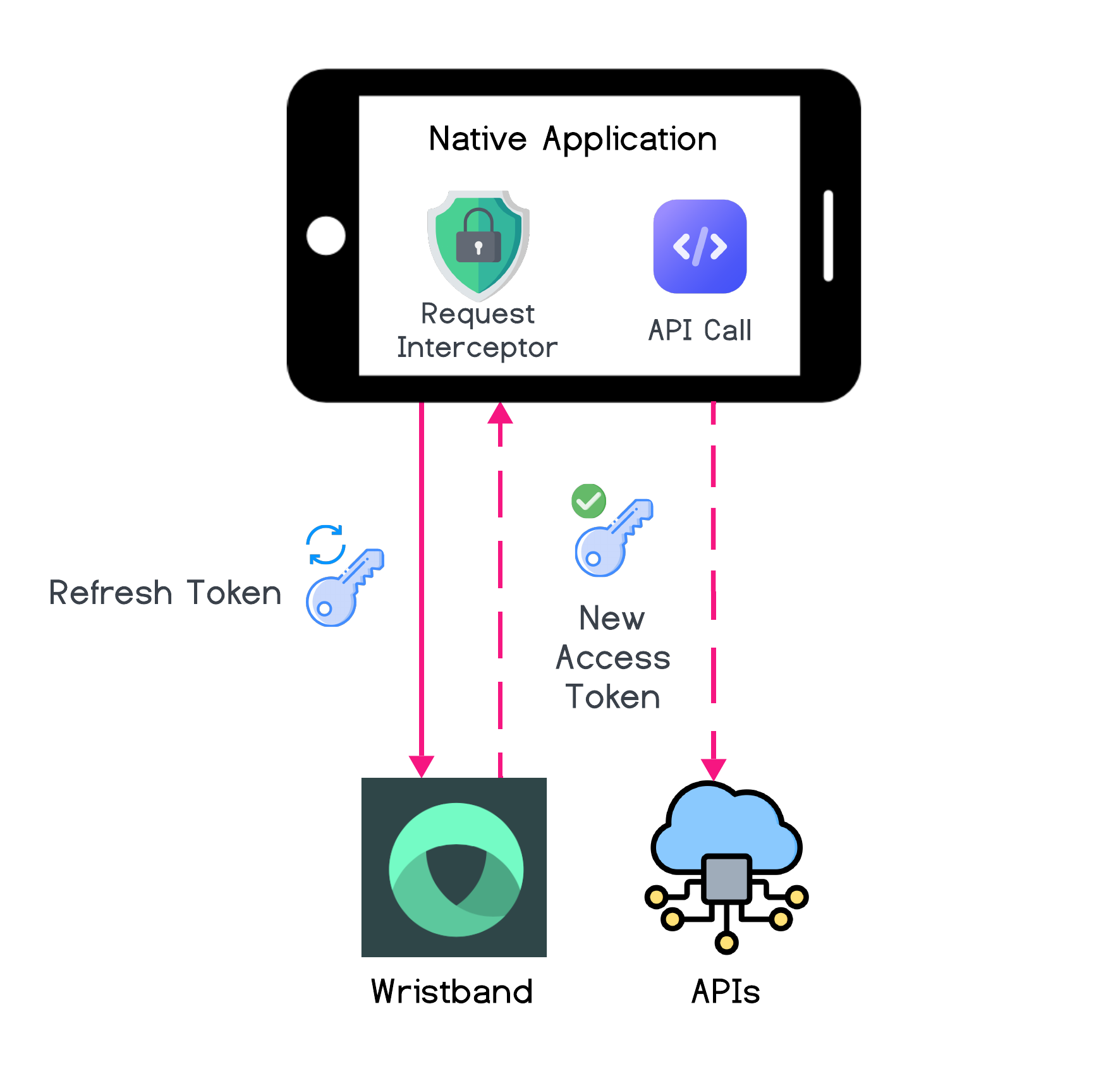
Refresh Token Flow for Native Integration
Additional Considerations
Access tokens should be refreshed slightly before their expiration time is reached in order to prevent situations where the access token expires while being processed by downstream API servers, resulting in a 401 being returned to the app. While the app should be able to recover from the 401 by refreshing the access token and retrying the request, it results in extra latency due to having to repeat the same request.
Refresh Token Rotation
Wristband supports refresh token rotation, a security feature that issues a new refresh token with every use, reducing the risk of token replay attacks. However, native apps inherently provide secure storage environments for tokens, such as encrypted keychains or secure storage APIs offered by the platform. This ensures that tokens are not exposed to malicious access within the app.
Because of these secure storage mechanisms, refresh token rotation is not required for native apps. Tokens remain secure as long as the app leverages proper storage methods and access controls provided by the operating system. To simplify implementation and reduce complexity, Wristband disables refresh token rotation by default for native app configurations.
Handling Refresh Failures
A failed token refresh in native apps indicates that the refresh token could not be used to obtain a new access token from Wristband. This can occur due to several reasons:
- The refresh token’s expiration time has been exceeded.
- The refresh token has been revoked.
- The refresh token has been tampered with and is no longer valid.
- An invalid request, such as incorrect client credentials.
- Network issues or server errors (e.g., 500 Internal Server Error).
Retrying Refresh Requests
For transient issues like network or server errors, it is advised to retry the refresh request to minimize disruption for the end user. We recommend implementing retry logic with a fixed or exponential backoff.
When the refresh token operation fails, the native app can handle it in various ways based on your UX and security requirements.
- Redirect to Login Without Destroying the Wristband Auth Session: Send the user to the Wristband login page without calling the Wristband logout endpoint. If the user still has a valid Wristband auth session, they will automatically be re-authenticated without having to enter their credentials.
- Full Logout and Re-authentication: Clean up any local session data from your app, then redirect the user to Wristband’s Logout Endpoint to terminate the Wristband auth session. This will force the user to re-authenticate the next time they try to access your application.
Updated about 1 month ago