🧪 Test Auth Middleware
Ensure that the auth middleware protects APIs and your frontend handles error responses.
Tests not working?
If you get stuck, contact us and our development team will work directly with you to get unblocked.
We'll perform a similar set of tests as previous steps in this guide to ensure the middleware works as expected.
1. Verify Redirect to Login When Unauthenticated
Verify that the middleware protects your Session endpoint.
Actions
- While logged out of your application, attempt to navigate directly to your React frontend's home page in the browser. For example:
http://localhost:3000/your-react-home-route
Verify Login Redirect
- Verify that the network requests show a
401
Unauthorized HTTP response coming from your Express server's Session endpoint. - Verify in the network requests that the browser redirects you to your Express server's Login endpoint.
- Verify that you land on either the Tenant Discovery Page or the Tenant Login Page of your test tenant (depending on you configure/manage your domains).
2. Verify Successful Login
Verify that the middleware is not breaking the login flow.
Actions
- While on the Tenant Login Page, enter your test user's email and password.
- Click the "Log In" button.
Verify React Frontend Access
- Verify that no errors occurred when submitting your credentials on the Tenant Login Page.
- Verify that you land on the home route in your React frontend.
- Verify that you have an application session cookie in your browser.
3. Verify Protected Resource API
Verify that the middleware protects some arbitrary API in your Express Server, similar to the Session endpoint.
Implement Test Endpoint and Component
For this test, make sure you have some test API implemented in your Express server that is protected by the auth middleware. It could be a simple API that just returns a 204 response.
// app.ts
import { authMiddleware } from './middleware';
...
// Test endpoint
app.get('/test', [authMiddleware], (req, res) => {
return res.status(204).send();
});
...
In your React frontend, add a test component that makes an API request to your test endpoint.
// test-component.tsx
import axios from 'axios';
import { isUnauthorized, redirectToLogin } from '@/utils/helpers';
export function ExampleComponent() {
const handleApiCall = async () => {
try {
const response = await axios.get(`<your-express-server-domain>/test`);
alert('Success!');
} catch (error: unknown) {
if (isUnauthorized(error)) {
redirectToLogin();
} else {
console.error('Unexpected error:', error);
alert('Something went wrong!');
}
}
};
return (
<button onClick={handleApiCall}>
Make API Call
</button>
);
}
Verify Successful API Call
First we'll make sure the happy path to your test endpoint works as expected.
Actions
- After successfully logging into your application, click the button in your test component to trigger the API call to your test endpoint.
Verify Success
- Verify that no errors occurred during the API call.
- Verify that a success message is displayed in the UI.
- Verify in the network requests in your browser that a
204 No Content
HTTP response status was returned to your frontend.
Verify Unauthenticated API Call
We'll need to simulate your application session cookie expiring in order to trigger a 401 Unauthorized
HTTP response status from the auth middleware.
Actions
- While still logged in to the application, manually delete the application session cookie from the browser.
- Click the button in your test component to trigger the API call to your test endpoint.
Verify Login Redirect
- Verify that the network requests show a
401
Unauthorized HTTP response coming from your Express server's test endpoint. - Verify in the network requests that the browser redirects you to your Express server's Login endpoint.
- Verify that you land on either the Tenant Discovery Page or the Tenant Login Page of your test tenant (depending on how you configured your domains).
If all tests succeeded, then your auth middleware is working as expected! 🎉
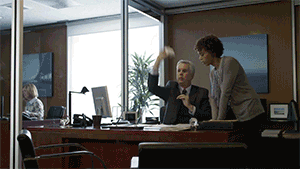
Unauthorized access? Not in YOUR routes!
Next, we'll implement a Cross Site Request Forgery (CSRF) middleware as another critical layer of defense for your application.
Updated 1 day ago